
Introduction to PHP
PHP: Hypertext Preprocessor (or simply PHP) is a server-side scripting language designed for Web development, but also used as a general-purpose programming language. It was originally created by Rasmus Lerdorf in 1994, the PHP reference implementation is now produced by The PHP Group. PHP originally stood for Personal Home Page, but it now stands for the recursive acronym PHP: Hypertext Preprocessor.
PHP code may be embedded into HTML code, or it can be used in combination with various web template systems, web content management systems, and web frameworks. PHP code is usually processed by a PHP interpreter implemented as a module in the web server or as a Common Gateway Interface (CGI) executable. The web server combines the results of the interpreted and executed PHP code, which may be any type of data, including images, with the generated web page. PHP code may also be executed with a command-line interface (CLI) and can be used to implement standalone graphical applications.
The standard PHP interpreter, powered by the Zend Engine, is free software released under the PHP License. PHP has been widely ported and can be deployed on most web servers on almost every operating system and platform, free of charge.
<?php
echo "My first PHP code line! :D";
?>
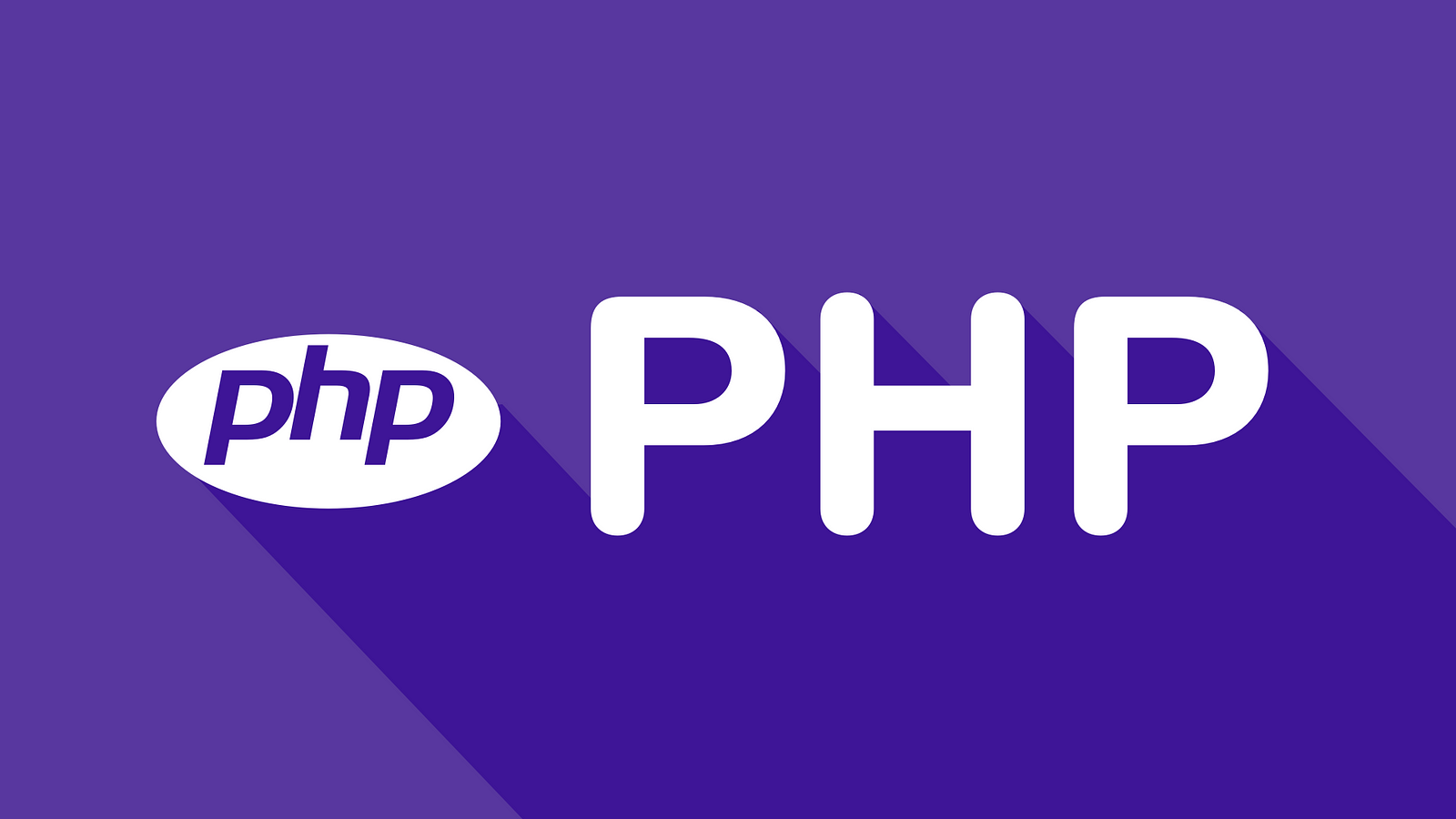
Basics things about PHP
When you learn something new, it is very important to remember basic things about the stuff you're trying to learn. You know, the stuff you quite often omit that are very important. Like:
- Your text file must be saved with ".php" extension. Not with ".php.txt" but with just ".php". This is achieved by choosing option "all files" which is found under field where you type name of the file when you save. Then you add at the end of file an extension.
- Every php file i.e code inside it, start with left arrow "<"
- followed by question mark "?"
- then you add to it a string "php" which is followed by one empty space
- which afterwards you can enter your php code.That how servers know it's a php code that it can executes it.
- After you done with coding, make one empty space you, then you should close it with question mark "?" followed with right arrow. ">"
So it goes something like this: <?php //yourPHPCodeGoesHere ?>
Ofcourse <?php
, your code
and ?>
don't have to be on the same line. You can break it!

Data types
PHP supports the following data types:
- String
- Integer
- Float (floating point numbers - also called double)
- Boolean
- Array
- Object
- NULL
- Resource
PHP String
A string is a sequence of characters, like "Hello world!".
A string can be any text inside quotes. You can use single or double quotes:
<?php
$x = "Hello world!";
$y = 'Hello world!';
echo $x;
echo "<br>";
echo $y;
?>
PHP Integer
An integer data type is a non-decimal number between -2,147,483,648 and 2,147,483,647.
Rules for integers:
- An integer must have at least one digit
- An integer must not have a decimal point
- An integer can be either positive or negative
- Integers can be specified in three formats: decimal (10-based), hexadecimal (16-based - prefixed with 0x) or octal (8-based - prefixed with 0)
In the following example $x is an integer. The PHP var_dump() function returns the data type and value:
<?php
$x = 5985;
var_dump($x);
?>
PHP Float
A float (floating point number) is a number with a decimal point or a number in exponential form.
In the following example $x is a float. The PHP var_dump() function returns the data type and value:
<?php
$x = 10.365;
var_dump($x);
?>
PHP Boolean
A Boolean represents two possible states: TRUE or FALSE.
Booleans are often used in conditional testing.
<?php
$x = true;
$y = false;
?>
PHP Array
An array stores multiple values in one single variable.
In the following example $cars is an array. The PHP var_dump() function returns the data type and value:
<?php
$names = array("Johnny","Tom","Loyd");
var_dump($names);
?>
PHP Object
An object is a data type which stores data and information on how to process that data.
In PHP, an object must be explicitly declared.
First we must declare a class of object. For this, we use the class keyword. A class is a structure that can contain properties and methods:
<?php
class Car {
function Car() {
$this->model = "VW";
}
}
// create an object
$herbie = new Car();
// show object properties
echo $herbie->model;
?>
PHP NULL Value
Null is a special data type which can have only one value: NULL.
A variable of data type NULL is a variable that has no value assigned to it.
If a variable is created without a value, it is automatically assigned a value of NULL.
Variables can also be emptied by setting the value to NULL:
<?php
$x = "Hello world!";
$x = null;
var_dump($x);
?>
PHP Resource
The special resource type is not an actual data type. It is the storing of a reference to functions and resources external to PHP.
A common example of using the resource data type is a database call.
If a variable is created without a value, it is automatically assigned a value of NULL.
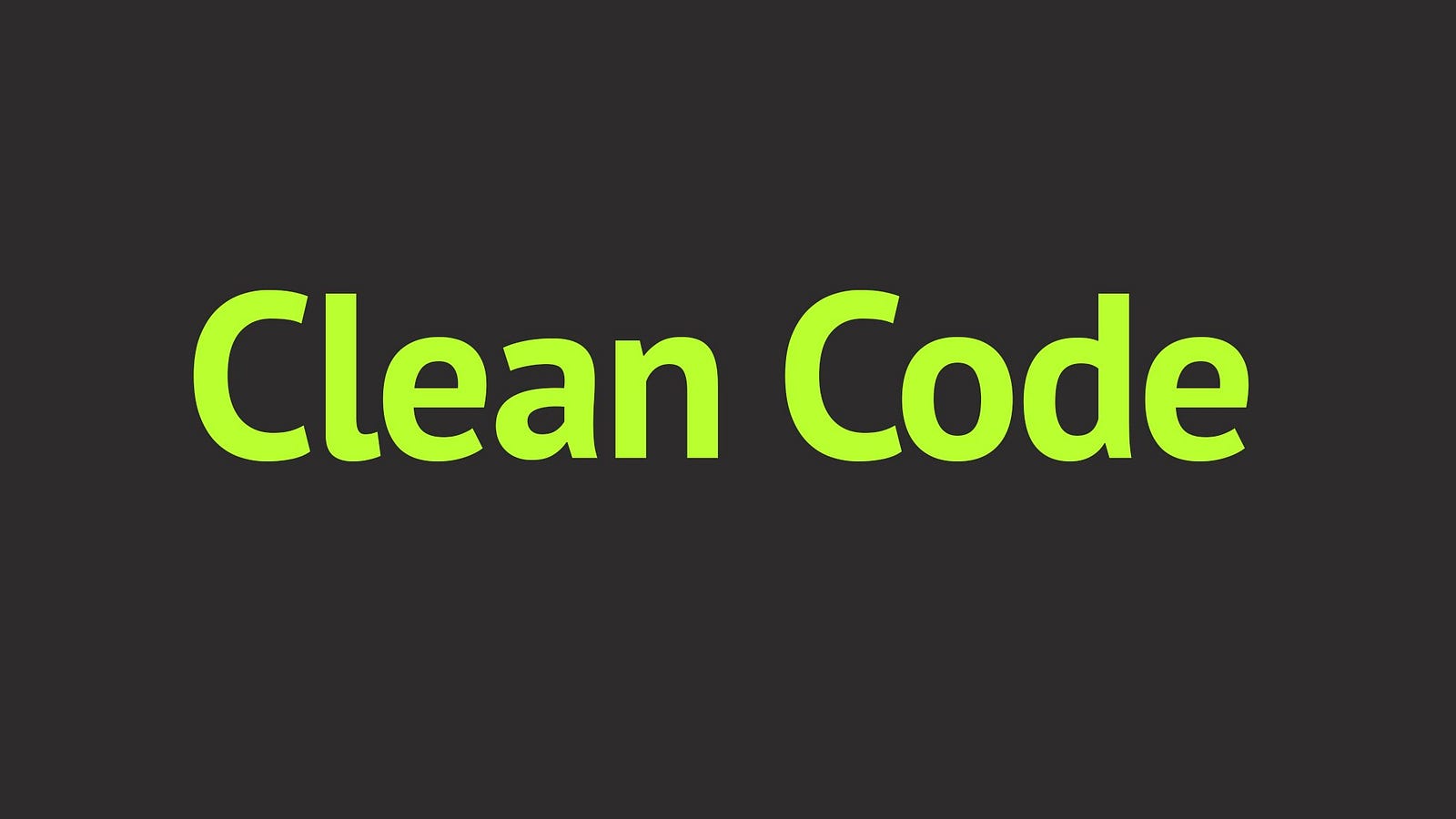
Variable scope
What is "variable scope":
Variable scope is known as its boundary within which it can be visible or accessed from code. In other words, it is the context within which a variable is defined. There are only two scopes available in PHP namely local and global scopes.
- Local variables (local scope)
- Global variables (special global scope)
- Static variables (local scope)
- Function parameters (local scope)
When a variable is accessed outside its scope it will cause PHP error Undefined Variable.
Local Scope Variables
A local scope is a restricted boundary of a variable within which code block it is declared. That block can be a function, class or any conditional span. The variable within this limited local scope is known as the local variable of that specific code block.
The following code block shows a PHP function. We have declared a variable $count inside this function. This variable is said to be a local variable of this function and it is in the local scope of the function block.
<?php
function calculate_count() {
$count = 5;
//will print 5; the value of local variable
echo $count++;
}
?>
Local variables will be destroyed once the end of the code block is reached. Hence the same named variables can be declared within different local scopes.
Global Scope Variables
As its name, the global scope provides widespread access to the variable declared in this scope. Variables in global scope can be accessed from anywhere from outside a function or class independent of its boundary.
PHP global variables can be defined by using global keyword. If we want to use global variables inside a function, we have to prefix the global keyword with the variable. The following code shows a code block to learn how to use the global keyword with a PHP variable to declared it as a global variable.
<?php
$count = 0;
function calculate_count() {
global $count;
// will print 0 and increment global variable
echo $count++ . "<br>";
}
calculate_count();
echo $count;
?>
PHP has a predefined superglobal variable called $GLOBALS. It is an associative array with the name of the variable as key and value as the array element. We can use this array variable to add an array of PHP variables in a global scope.
Let us change the above example with the global keyword by using $GLOBALS superglobal to access the variable in global scope.
<?php
$count = 0;
function calculate_count() {
// will print 0 and increment global variable declared outside function
echo $GLOBALS["count"]++ . "<br/>";
}
calculate_count();
echo $count;
?>
Static Variables (local scope)
A static variable is again a variable with local scope. But the difference with the regular local variable is that it is not destroyed outside the scope boundary. A variable can be defined by using the ‘static’ keyword inside a function.
A static variable does not lose its value when the program execution goes past the scope boundary. But it can be accessed only within that boundary. Let me demonstrate it using the following example code:
<?php
function counter(){
static $count = 0;
echo $count;
$count++;
}
?>
The above counter function has the static variable ‘count’ declared within its local scope. When the function execution is complete, the static count variable still retains its value for further computation. Every time the counter function is called, the value of count is incremented. The count value is initialized only once on the first call.
Function Parameters (Local Scope)
Function parameters (arguments) are local variables defined within the local scope of the function on which it is used as the argument.
Scope and File Includes:
The boundary of file includes does not demarcate the scope of variables. The scope of a variable is only governed by the function block and not based on the file include. A variable can be declared in a PHP file and used in another file by using ‘include’ or ‘require’ that file.
Function Inside Function or Class:
Remember that the scope in PHP is governed by a function block. Any new function declared anywhere starts a new scope. If an anonymous function is defined inside another function, the anonymous function has its own local scope.
<?php
function foo() {
$fruit = 'apple';
$bar = function () {
// $fruit cannot be accessed inside here
$animal = 'lion';
};
// $animal cannot be accessed outside here
}
?>
In the above example code, $fruit variable is restricted to the outer function and its scope does not span inside the anonymous inner function. The same way, $animal which is declared inside is not accessible in the outer function as its scope boundary is restricted to the inner function.
General Note:
Whenever you want to use a variable in a different scope, the way in and way out is by passing as an argument. Do not use global scoped variable for such trivial use cases.
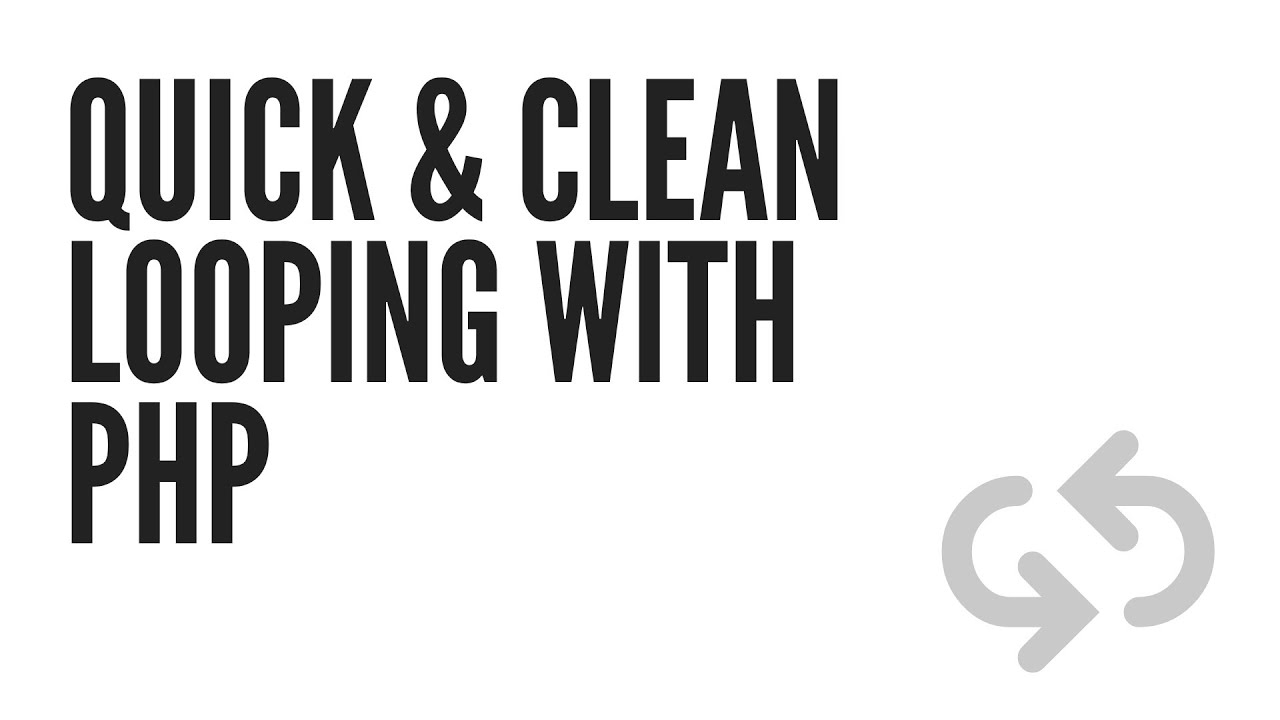
Loops
Loops are used to execute the same block of code again and again, until a certain condition is met. The basic idea behind a loop is to automate the repetitive tasks within a program to save the time and effort. PHP supports four different types of loops:
- While — loops through a block of code until the condition is evaluate to true.
- Do…While — the block of code executed once and then condition is evaluated. If the condition is true the statement is repeated as long as the specified condition is true.
- For — loops through a block of code until the counter reaches a specified number.
- Foreach — loops through a block of code for each element in an array.
While Loop
The while statement will loops through a block of code until the condition in the while statement evaluate to true.
<?php
while(condition){
// Code to be executed
}
?>
The example below define a loop that starts with $i=1
. The loop will continue to run as long as $i is less than or equal to 3. The $i
will increase by 1 each time the loop runs:
<?php
$i = 1;
while($i <= 3){
$i++;
echo "The number is " . $i . " <br>";
}
?>
Do…While Loop
The do-while loop is a variant of while loop, which evaluates the condition at the end of each loop iteration. With a do-while loop the block of code executed once, and then the condition is evaluated, if the condition is true, the statement is repeated as long as the specified condition evaluated to is true.
<?php
do{
// Code to be executed
}
while(condition);
?>
The following example define a loop that starts with $i=1. It will then increase $i with 1, and print the output. Then the condition is evaluated, and the loop will continue to run as long as $i is less than, or equal to 3.
<?php
$i = 1;
do{
$i++;
echo "The number is " . $i . "<br>";
}
while($i <= 3);
?>
Difference between While and Do…While Loop
The while loop differs from the do-while loop in one important way — with a while loop, the condition to be evaluated is tested at the beginning of each loop iteration, so if the conditional expression evaluates to false, the loop will never be executed.
With a do-while loop, on the other hand, the loop will always be executed once, even if the conditional expression is false, because the condition is evaluated at the end of the loop iteration rather than the beginning.
For Loop
The for loop repeats a block of code until a certain condition is met. It is typically used to execute a block of code for certain number of times.
for(initialization; condition; increment){
// Code to be executed
}
The parameters of for loop have following meanings:
- initialization — it is used to initialize the counter variables, and evaluated once unconditionally before the first execution of the body of the loop.
- condition — in the beginning of each iteration, condition is evaluated. If it evaluates to true, the loop continues and the nested statements are executed. If it evaluates to false, the execution of the loop ends.
- increment — it updates the loop counter with a new value. It is evaluate at the end of each iteration.
The example below defines a loop that starts with $i=1. The loop will continued until $i is less than, or equal to 5. The variable $i will increase by 1 each time the loop runs:
<?php
for($i=1; $i<=3; $i++){
echo "The number is " . $i . "<br>";
}
?>
ForEach Loop
The foreach loop is used to iterate over arrays.
<?php
foreach($array as $value){
// Code to be executed
}
?>
The following example demonstrates a loop that will print the values of the given array:
<?php
$colors = array("Red", "Green", "Blue");
// Loop through colors array
foreach($colors as $value){
echo $value . " <br>";
}
?>
There is one more syntax of foreach loop, which is extension of the first.
<?php
foreach($array as $key => $value){
// Code to be executed
}
?>
Example:
<?php
$superhero = array(
"name" => "Peter Parker",
"email" => "peterparker@mail.com",
"age" => 18
);
// Loop through superhero array
foreach($superhero as $key => $value){
echo $key . " : " . $value . "<br>";
}
?>

Functions in PHP
Besides PHP's built-in functions, there's also user defined functions.
User defined functions
Their main qualities are:
- A function is a block of statements that can be used repeatedly in a program.
- A function will not execute immediately when a page loads.
- A function will be executed by a call to the function.
- Any valid PHP code may appear inside a function, even other functions and class definitions.
- Function can accept arguments and can also return a value "computed" by it.
- A user-defined function declaration starts with the word function.
Function names follow the same rules as other labels in PHP. A valid function name starts with a letter or underscore, followed by any number of letters, numbers, or underscores. As a regular expression, it would be expressed thus: [a-zA-Z_\x7f-\xff][a-zA-Z0-9_\x7f-\xff]*.
Syntax is something like this:
<?php
function foo($arg_1, $arg_2, /* ..., */ $arg_n){
echo "Example function.\n";
return $retval;
}
?>
In the example below, we create a function named "writeMsg()". The opening curly brace ( { ) indicates the beginning of the function code and the closing curly brace ( } ) indicates the end of the function. The function outputs "Hello world!". To call the function, just write its name:
<?php
function writeMsg() {
echo "Hello world!";
}
writeMsg(); // call the function
?>
PHP Function Arguments
Information can be passed to functions through arguments. An argument is just like a variable.
Arguments are specified after the function name, inside the parentheses. You can add as many arguments as you want, just separate them with a comma.
The following example has a function with one argument ($fname). When the familyName() function is called, we also pass along a name (e.g. Jani), and the name is used inside the function, which outputs several different first names, but an equal last name:
<?php
function familyName($fname) {
echo "$fname Refsnes.<br>";
}
familyName("Jani");
familyName("Hege");
familyName("Stale");
familyName("Kai Jim");
familyName("Borge");
?>
The following example has a function with two arguments ($fname and $year):
<?php
function familyName($fname, $year) {
echo "$fname Refsnes. Born in $year <br>";
}
familyName("Hege", "1975");
familyName("Stale", "1978");
familyName("Kai Jim", "1983");
?>
PHP Default Argument Value
The following example shows how to use a default parameter. If we call the function setHeight() without arguments it takes the default value as argument:
<?php
function setHeight($minheight = 50) {
echo "The height is : $minheight <br>";
}
setHeight(350);
setHeight(); // will use the default value of 50
setHeight(135);
setHeight(80);
?>
PHP Functions - Returning values
To let a function return a value, use the return statement:
<?php
function sum($x, $y) {
$z = $x + $y;
return $z;
}
echo "5 + 10 = " . sum(5, 10) . "<br>";
echo "7 + 13 = " . sum(7, 13) . "<br>";
echo "2 + 4 = " . sum(2, 4);
?>

Reference
Sources: